JavaScript’s Temporal API: the future of date time handling!
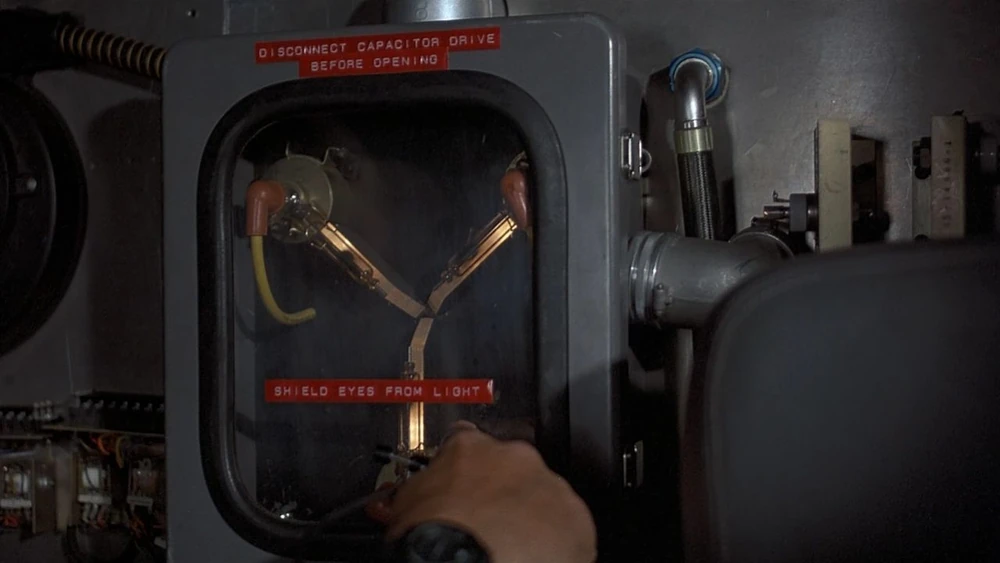
Ah, the JavaScript Date object. It just doesn't cut it, does it? Working with dates can be daunting or maddening! So rather than using the Date object, we've been resorting to libraries (like moment.js or date-fns) that tackle the complexity for us. We're getting at a comfortable space right now, with the Temporal API!
It's not widely supported right now (experimental stage), but close to being finalized and adopted by major browsers!
Let’s warm up the flux capacitor and dive into the Temporal API. Spoiler: it’s not just about better date formatting (though, that’s a big part of it).
The Problem with Date
If you’ve worked with the Date object in JavaScript for more than five minutes, you’ve likely wanted to punch a dolphin in the mouth. Between time zones, leap years, and the general confusion of unexpected results, it’s been a wild ride. The Date object is like that one kitchen drawer filled with mismatched utensils—functional but chaotic.
That's why libraries stepped in to tidy things up. It took some time, but now we're on the verge of getting a useful API to handle those pesky date and time situations.
Temporal: First Impressions
Unlike the Date object, Temporal is straightforward and specific. Instead of relying on relative and implicit assumptions, Temporal uses explicit types for each time-related concept. You’re not just slapping together a date and hoping for the best anymore.
Let’s look at a simple example:
const now = Temporal.Now.plainDateISO();
console.log(now); // 2024-12-31
Easy, right? No time zone confusion, no guesswork—just clarity. It’s ISO 8601 (regardless of cultural formatting preference). So far so good. But Temporal doesn’t stop there. It handles everything from dates to times, durations, and even calendars.
Explicit is Better Than Implicit
The first thing you’ll notice about Temporal is that it doesn’t try to “help” you in the same way Date does—by making guesses about what you mean. Want to create a specific moment in time? You better be sure about exactly what you mean.
const someDate = Temporal.PlainDate.from('2024-12-31');
Want to try and flip the days and months? No sir! The API returns an error, explaining exactly what went wrong ("Uncaught RangeError: can't parse date-time: month must be a number from 1 to 12"). No more guessing! Why Date returns an "Invalid Date" error. It's the little things, right?
Having Fun with Time Zones (Just Kidding)
I am very fortunate to (knock on wood) never really had to deal with time zone-related bugs. And given some time, I never have to, with Temporal coming to the rescue again. 💪
const zoned = Temporal.ZonedDateTime.from('2024-12-31T15:30:00[Europe/Amsterdam]');
console.log(zoned.toString()); // 2024-12-31T15:30:00+01:00[Europe/Amsterdam]
Built in, clear handling of timezones! Temporal even keeps track of daylight saving time for you, so you can stop worrying about whether your app breaks when the clocks change over the course of weeks across the globe. Imagine that.
Durations: More Than Just Counting the Days
Temporal also makes working with durations—something we all do at some point—a breeze:
const duration = Temporal.Duration.from({ days: 7, hours: 5 });
const newDate = Temporal.PlainDate.from('2024-12-31').add(duration);
console.log(newDate.toString()); // 2025-01-07
This has to be one of the features I love most: it's so flexible and readable at the same time!
The Catch (for now)
Now (not a pun), before you get too excited, as I said: the Temporal API is not yet natively available in every browser as of writing this. So you may still need polyfills or tooling support. That's fine too, been there, done that!
Don’t let it stop you. Temporal is the future, and like all good things in JavaScript, it will eventually become the standard.
I've been playing with the Temporal API in Firefox Nightly, but it's likely to be available on more browsers in Canaries, Nightlies or behind feature flags. I recommend trying it out, but since it's still experimental, might be subject to changes. I highly doubt it will be a complete overhaul, but it's definitely not ready for your production environments!